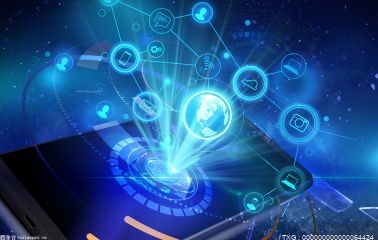
首先讲讲版本问题,如果使用vue-cli2
模版搭建的基础项目,注意,如果使用vue
版本是2,当你你默认安装vuex
肯定是4.x
版本了,这里需要注意的是,你要降低vuex
版本到3.x
版本,不然store
挂载不到vue
上
【资料图】
当我们修改数据,只能通过mutation
修改state
import Vue from "vue"import Vuex from "vuex"Vue.use(Vuex)export const store = new Vuex.Store({ state: { data: [] }, mutations: { storeData (state, payload) { state.data = state.data.concat(payload) } }})
在页面中
import { mockFeatchData } from "@/mock"export default { name: "HelloWorld", data () { return { msg: "Welcome to Your Vue.js App" } }, computed: { ...mapState({ dataList: state => state.data }) }, methods: { handleData () { mockFeatchData().then(res => { this.$store.commit("storeData", res) }) } }}
我们修改数据就是$store.commit("eventName", payload)
,当我们触发commit
时,实际上是已经在异步请求回调里获取了数据。
但是官方在描述mutation
有这么说,mutation
内部必须是同步函数,异步会导致内部状态难以追踪,devtool
难以追踪state
的状态
...mutations: { storeData (state, payload) { mockFeatchData().then((res) => { console.log(res) state.data = state.data.concat(res) }) }}
也就是说上面这段代码,当我们在mutations
中的storeData
中使用了异步函数,我们在$store.commit("storeData")
时,很难追踪state
的状态,因为在触发commit事件时,异步的回调函数
不知道什么时候执行,所以难以追踪。
mutations
是同步事务,假设在mutations
有多个异步的调用,你很难确定这些异步哪些先执行,很难追踪state
的变化,所以也给调试带来了一定难度
话说回来,这么写也确实是可以做到更新state的值
,如果我不用vuetool
这个工具,貌似也没毛病
既然mutations
是同步的事情,那么异步官方就使用了actions
方案
actions
里面可以做异步操作,但是并不是直接修改数据,提交的是mutations
里面的方法
mutations: { storeData (state, payload) { state.data = state.data.concat(payload) }},actions: { setStoreData ({ commit }) { mockFeatchData().then((res) => { commit("storeData", res) }) } }
在页面中就是这样触发actions
的
methods: { handleData () { this.$store.dispatch("setStoreData") } }
我们把异步操作放在了actions
的方法里面,你会发现mockFeatchData
这是一个异步操作后的结果,然后通过commit
传给了mutations
中
在actions
执行异步操作,将结果给了mutations
,mutations
中同步修改状态state
,使得actions
的操作在mutations
中有记录。
在actions
中也可以有多个异步操作
mutations: { storeData (state, payload) { state.data = state.data.concat(payload) }, storeText (state, payload) { state.text = payload } }, actions: { setStoreData ({ commit }) { mockFeatchData().then((res) => { console.log(res, "111") commit("storeData", res) }) }, setStoreText ({ dispatch, commit }, payload) { dispatch("setStoreData").then(() => { console.log(222) commit("storeText", payload) }) } }复制代码
页面上是这样触发actions
的
handleText () { this.$store.dispatch("setStoreText", `hello,${Math.random()}`) }复制代码
这里我们也可以用对象的方式
handleText () { this.$store.dispatch({ type: "setStoreText", payload: `hello,${Math.random()}`})复制代码
不过此时注意actions
中获取值需要解构才行
setStoreText ({ dispatch, commit }, {payload}) { dispatch("setStoreData").then(() => { console.log(222, payload) commit("storeText", payload) })}复制代码
在actions可以dispatch
另一个异步的操作,也就是等一个任务完成了后,可以执行另一个commit
看到这里貌似这里有点明白,为啥所有的异步操作放在actions
里面了,mutation
只负责修改state
,所有异步操作产生的副作用的结果都统统交给了mutation
,这样很好保证devtool
了对数据的追踪。